Overview
Getting Started
We provide a fully restful API with direct and powerful access to a vast array of features. Companies, developers and researchers can incorporate our innovative services directly into software and services.To make a Dynu REST request, follow the three steps below.
Note: You can run the examples listed here directly as-is. However, when creating your own application, be sure to update the OAuth keys in your code with those assigned to your own account.
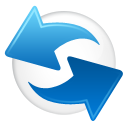
You may also review the IP update protocol to control the state of dynamic DNS service.
1
Obtain OAuth keys
The OAuth keys consists of
client_id
and secret
.
The keys uniquely identify your account and you can create, reset or clear the keys in the
API Credentials area of the control panel.Tip: See how the OAuth keys work by running the example in the next step—the example uses a set of dummy keys so you can easily make your first call by pasting the example as-is into a terminal window.
2
Get an Access Token
Make a
/token
call using your application's OAuth keys for the basic authentication values (the keys are the values of your client_id
and secret
).
In the request body, set grant_type
to client_credentials
.
When you run the command, Dynu generates and returns a new access token.Tip: If you're using cURL, supply the OAuth values like this:
-u {Client-Id}:{Secret}
. If you're using the Postman tool, enter the client_id
and secret
values on the Basic Auth tab using the client_id
as the username and the secret as the password. Additionally, on the x-www-form-unlencoded tab, specify a grant_type
of client_credentials
.Example access token request
curl -v https://api.dynu.com/v1/oauth2/token \
-H "Accept: application/json" \
-H "Accept-Language: en_US" \
-u "EOJ2S-Z6OoN_le_KS1d75wsZ6y0SFdVsY9183IvxFyZp:EClusMEUk8e9ihI7ZdVLF5cZ6y0SFdVsY9183IvxFyZp" \
-d "grant_type=client_credentials"
-H "Accept: application/json" \
-H "Accept-Language: en_US" \
-u "EOJ2S-Z6OoN_le_KS1d75wsZ6y0SFdVsY9183IvxFyZp:EClusMEUk8e9ihI7ZdVLF5cZ6y0SFdVsY9183IvxFyZp" \
-d "grant_type=client_credentials"
Tip: If you're using Windows, we recommend you make cURL calls using a Bash shell. If you're not using cURL calls, set the
content-type
to application/x-www-form-urlencoded
for this request.Sample response:
{
"scope": "https://api.dynu.com/v1/Domain/.* https://api.dynu.com/v1/DNS/.* https://api.dynu.com/v1/Email/.*",
"access_token": "<Access-Token>",
;"token_type": "Bearer",
"expires_in": 28800
}
"scope": "https://api.dynu.com/v1/Domain/.* https://api.dynu.com/v1/DNS/.* https://api.dynu.com/v1/Email/.*",
"access_token": "<Access-Token>",
;"token_type": "Bearer",
"expires_in": 28800
}
Note: The access token is valid for the number of seconds specified in the
expires_in
response value. You must have a valid access token to make API requests—request a new token when the current one expires.
3
Make an API call
With a valid access token in hand, you're ready to make a request to a REST interface. Below is call to obtain a list of domain names which have DNS service. The simple request uses only the required input fields.
The access token is an OAuth bearer token, and is included in the header of your requests with the following syntax:
Authorization: Bearer <Access-Token>
.Important: You must supply a valid access token to complete this request (generate a valid token using the example call above).
Example /dns/domains request
curl -v https://api.dynu.com/v1/domain/domains \
-H "Content-Type: application/json" \
-H "Authorization: Bearer <token>"
-H "Content-Type: application/json" \
-H "Authorization: Bearer <token>"
If the call is successful, Dynu returns a list of domain names along with their basic details. You can find the same list of domain names in the control panel.
Response
[
{
"id": 1,
"user_id": 11,
"name": "example.com",
"unicode_name": "example.com",
"token": "domain-token",
"state": "complete",
"language":null,
"lockable": false,
"auto_renew": true,
"whois_protected": false,
"expires_on": "2018-07-20T15:01:13",
"created_at": "2015-07-28T15:01:13",
"updated_at": "2015-09-10T13:03:15"
},
{
"id": 2,
"user_id": 11,
"name": "example.org",
"unicode_name": "example.org",
"token": "domain-token",
"state": "complete",
"language":null,
"lockable":false,
"auto_renew": true,
"whois_protected": false,
"expires_on": "2016-08-05T23:09:12",
"created_at": "2015-08-05T23:09:12",
"updated_at": "2015-09-10T13:05:38"
}
]
{
"id": 1,
"user_id": 11,
"name": "example.com",
"unicode_name": "example.com",
"token": "domain-token",
"state": "complete",
"language":null,
"lockable": false,
"auto_renew": true,
"whois_protected": false,
"expires_on": "2018-07-20T15:01:13",
"created_at": "2015-07-28T15:01:13",
"updated_at": "2015-09-10T13:03:15"
},
{
"id": 2,
"user_id": 11,
"name": "example.org",
"unicode_name": "example.org",
"token": "domain-token",
"state": "complete",
"language":null,
"lockable":false,
"auto_renew": true,
"whois_protected": false,
"expires_on": "2016-08-05T23:09:12",
"created_at": "2015-08-05T23:09:12",
"updated_at": "2015-09-10T13:05:38"
}
]
Ping
1
Ping to verify connectivity to our platform
GET /v1/ping
ExamplePing to get a
Success
response.
curl -v https://api.dynu.com/v1/ping \
-H "Content-Type: application/json" \
-H "Authorization: Bearer <token>"
Response-H "Content-Type: application/json" \
-H "Authorization: Bearer <token>"
Responds with HTTP/1.1 200 OK if successful, returns the response.
{
"type": "Success",
"message": ""
}
"type": "Success",
"message": ""
}
Domain Registration
Domains
1
Get a domain
GET /v1/domain/get/:domain
Parameters
Name | Type | Description |
---|---|---|
:domain | string, integer | The domain name or id |
Get the domain with ID
1
.
curl -v https://api.dynu.com/v1/domain/get/1 \
-H "Content-Type: application/json" \
-H "Authorization: Bearer <token>"
Get the domain -H "Content-Type: application/json" \
-H "Authorization: Bearer <token>"
example.com
.
curl -v https://api.dynu.com/v1/domain/get/example.com \
-H "Content-Type: application/json" \
-H "Authorization: Bearer <token>"
Response-H "Content-Type: application/json" \
-H "Authorization: Bearer <token>"
Responds with HTTP/1.1 200 OK if successful, returns the domain.
{
"id": 1,
"user_id": 11,
"name": "example.com",
"unicode_name": "example.com",
"token": "domain-token",
"state": "complete",
"language":null,
"lockable": false,
"auto_renew": true,
"whois_protected": false,
"expires_on": "2018-07-20T15:01:13",
"created_at": "2015-07-28T15:01:13",
"updated_at": "2015-09-10T13:03:15"
}
"id": 1,
"user_id": 11,
"name": "example.com",
"unicode_name": "example.com",
"token": "domain-token",
"state": "complete",
"language":null,
"lockable": false,
"auto_renew": true,
"whois_protected": false,
"expires_on": "2018-07-20T15:01:13",
"created_at": "2015-07-28T15:01:13",
"updated_at": "2015-09-10T13:03:15"
}
2
List domains
List all domains.
GET /v1/domain/domains
Example
curl -v https://api.dynu.com/v1/domain/domains \
-H "Content-Type: application/json" \
-H "Authorization: Bearer <token>"
Response-H "Content-Type: application/json" \
-H "Authorization: Bearer <token>"
Responds with HTTP/1.1 200 OK if successful.
[
{
"id": 1,
"user_id": 11,
"name": "example.com",
"unicode_name": "example.com",
"token": "domain-token",
"state": "complete",
"language":null,
"lockable": false,
"auto_renew": true,
"whois_protected": false,
"expires_on": "2018-07-20T15:01:13",
"created_at": "2015-07-28T15:01:13",
"updated_at": "2015-09-10T13:03:15"
},
{
"id": 2,
"user_id": 11,
"name": "example.org",
"unicode_name": "example.org",
"token": "domain-token",
"state": "complete",
"language":null,
"lockable":false,
"auto_renew": true,
"whois_protected": false,
"expires_on": "2016-08-05T23:09:12",
"created_at": "2015-08-05T23:09:12",
"updated_at": "2015-09-10T13:05:38"
}
]
{
"id": 1,
"user_id": 11,
"name": "example.com",
"unicode_name": "example.com",
"token": "domain-token",
"state": "complete",
"language":null,
"lockable": false,
"auto_renew": true,
"whois_protected": false,
"expires_on": "2018-07-20T15:01:13",
"created_at": "2015-07-28T15:01:13",
"updated_at": "2015-09-10T13:03:15"
},
{
"id": 2,
"user_id": 11,
"name": "example.org",
"unicode_name": "example.org",
"token": "domain-token",
"state": "complete",
"language":null,
"lockable":false,
"auto_renew": true,
"whois_protected": false,
"expires_on": "2016-08-05T23:09:12",
"created_at": "2015-08-05T23:09:12",
"updated_at": "2015-09-10T13:05:38"
}
]
3
Cancel a domain
Cancel the given domain from your account.
DELETE /v1/domain/cancel/:domain
Please note that for domains which are registered with Dynu for over 5 days this will not cancel the domain registration.Parameters
Name | Type | Description |
---|---|---|
:domain | string, integer | The domain name or id |
Cancel the domain with ID
1
.
curl -v https://api.dynu.com/v1/domain/cancel/1 \
-H "Content-Type: application/json" \
-H "Authorization: Bearer <token>"
Cancel the domain -H "Content-Type: application/json" \
-H "Authorization: Bearer <token>"
example.com
.
curl -v https://api.dynu.com/v1/domain/cancel/example.com \
-H "Content-Type: application/json" \
-H "Authorization: Bearer <token>"
Response-H "Content-Type: application/json" \
-H "Authorization: Bearer <token>"
Responds with HTTP/1.1 200 OK if successful with the state being 'Cancelled'.
{
"id": 1,
"user_id": 11,
"name": "example.com",
"unicode_name": "example.com",
"token": "domain-token",
"state": "Cancelled",
"language":null,
"lockable": false,
"auto_renew": true,
"whois_protected": false,
"expires_on": "2018-07-20T15:01:13",
"created_at": "2015-07-28T15:01:13",
"updated_at": "2015-09-10T13:03:15"
}
"id": 1,
"user_id": 11,
"name": "example.com",
"unicode_name": "example.com",
"token": "domain-token",
"state": "Cancelled",
"language":null,
"lockable": false,
"auto_renew": true,
"whois_protected": false,
"expires_on": "2018-07-20T15:01:13",
"created_at": "2015-07-28T15:01:13",
"updated_at": "2015-09-10T13:03:15"
}
Auto-Renewal
1
Enable auto-renewal for a domain name
Get /v1/domain/autorenewal_enable/:domain
Parameters
Name | Type | Description |
---|---|---|
:domain | string, integer | The domain name or id |
Enable auto-renewal for the domain
example.com
:
curl -v https://api.dynu.com/v1/domain/autorenewal_enable/example.com \
-H "Content-Type: application/json" \
-H "Authorization: Bearer <token>"
Response-H "Content-Type: application/json" \
-H "Authorization: Bearer <token>"
Responds with HTTP/1.1 200 OK if successful, returns the domain.
{
"id": 1,
"user_id": 11,
"name": "example.com",
"unicode_name": "example.com",
"token": "domain-token",
"state": "Complete",
"language":null,
"lockable": false,
"auto_renew": true,
"whois_protected": false,
"expires_on": "2018-07-20T15:01:13",
"created_at": "2015-07-28T15:01:13",
"updated_at": "2015-09-10T13:03:15"
}
"id": 1,
"user_id": 11,
"name": "example.com",
"unicode_name": "example.com",
"token": "domain-token",
"state": "Complete",
"language":null,
"lockable": false,
"auto_renew": true,
"whois_protected": false,
"expires_on": "2018-07-20T15:01:13",
"created_at": "2015-07-28T15:01:13",
"updated_at": "2015-09-10T13:03:15"
}
2
Disable auto-renewal for a domain name
Get /v1/domain/autorenewal_disable/:domain
Parameters
Name | Type | Description |
---|---|---|
:domain | string, integer | The domain name or id |
Disable auto-renewal for the domain
example.com
:
curl -v https://api.dynu.com/v1/domain/autorenewal_disable/example.com \
-H "Content-Type: application/json" \
-H "Authorization: Bearer <token>"
Response-H "Content-Type: application/json" \
-H "Authorization: Bearer <token>"
Responds with HTTP/1.1 200 OK if successful, returns the domain.
{
"id": 1,
"user_id": 11,
"name": "example.com",
"unicode_name": "example.com",
"token": "domain-token",
"state": "Complete",
"language":null,
"lockable": false,
"auto_renew": false,
"whois_protected": false,
"expires_on": "2018-07-20T15:01:13",
"created_at": "2015-07-28T15:01:13",
"updated_at": "2015-09-10T13:03:15"
}
"id": 1,
"user_id": 11,
"name": "example.com",
"unicode_name": "example.com",
"token": "domain-token",
"state": "Complete",
"language":null,
"lockable": false,
"auto_renew": false,
"whois_protected": false,
"expires_on": "2018-07-20T15:01:13",
"created_at": "2015-07-28T15:01:13",
"updated_at": "2015-09-10T13:03:15"
}
Lock/Unlock
1
Lock/Unlock a domain name
Get /v1/domains/lock/:domain
Parameters
Name | Type | Description |
---|---|---|
:domain | string, integer | The domain name or id |
Lock the domain name
example.com
:
curl -v https://api.dynu.com/v1/domain/lock/example.com \
-H "Content-Type: application/json" \
-H "Authorization: Bearer <token>"
Response-H "Content-Type: application/json" \
-H "Authorization: Bearer <token>"
Responds with HTTP/1.1 200 OK if successful, returns the domain.
{
"id": 1,
"user_id": 11,
"name": "example.com",
"unicode_name": "example.com",
"token": "domain-token",
"state": "Complete",
"language":null,
"lockable": true,
"auto_renew": true,
"whois_protected": false,
"expires_on": "2018-07-20T15:01:13",
"created_at": "2015-07-28T15:01:13",
"updated_at": "2015-09-10T13:03:15"
}
"id": 1,
"user_id": 11,
"name": "example.com",
"unicode_name": "example.com",
"token": "domain-token",
"state": "Complete",
"language":null,
"lockable": true,
"auto_renew": true,
"whois_protected": false,
"expires_on": "2018-07-20T15:01:13",
"created_at": "2015-07-28T15:01:13",
"updated_at": "2015-09-10T13:03:15"
}
2
Unlock a domain name
Get /v1/domain/unlock/:domain
Parameters
Name | Type | Description |
---|---|---|
:domain | string, integer | The domain name or id |
Unlock the domain
example.com
:
curl -v https://api.dynu.com/v1/domain/unlock/example.com \
-H "Content-Type: application/json" \
-H "Authorization: Bearer <token>"
Response-H "Content-Type: application/json" \
-H "Authorization: Bearer <token>"
Responds with HTTP/1.1 200 OK if successful, returns the domain.
{
"id": 1,
"user_id": 11,
"name": "example.com",
"unicode_name": "example.com",
"token": "domain-token",
"state": "Complete",
"language":null,
"lockable": false,
"auto_renew": false,
"whois_protected": false,
"expires_on": "2018-07-20T15:01:13",
"created_at": "2015-07-28T15:01:13",
"updated_at": "2015-09-10T13:03:15"
}
"id": 1,
"user_id": 11,
"name": "example.com",
"unicode_name": "example.com",
"token": "domain-token",
"state": "Complete",
"language":null,
"lockable": false,
"auto_renew": false,
"whois_protected": false,
"expires_on": "2018-07-20T15:01:13",
"created_at": "2015-07-28T15:01:13",
"updated_at": "2015-09-10T13:03:15"
}
Name Servers
1
Get name servers for a domain name
Get /v1/domain/name_servers/:domain
Parameters
Name | Type | Description |
---|---|---|
:domain | string, integer | The domain name or id |
Get name servers for the domain
example.com
:
curl -v https://api.dynu.com/v1/domain/name_servers/example.com \
-H "Content-Type: application/json" \
-H "Authorization: Bearer <token>"
Response-H "Content-Type: application/json" \
-H "Authorization: Bearer <token>"
Responds with HTTP/1.1 200 OK if successful, returns the name servers.
{
"name servers": [
"NS1.DYNU.COM",
"NS2.DYNU.COM",
"NS3.DYNU.COM",
"NS4.DYNU.COM",
"NS5.DYNU.COM"
]
}
"name servers": [
"NS1.DYNU.COM",
"NS2.DYNU.COM",
"NS3.DYNU.COM",
"NS4.DYNU.COM",
"NS5.DYNU.COM"
]
}
2
Add name servers for a domain name
POST /v1/domain/name_server_add/:domain
Parameters
Name | Type | Description |
---|---|---|
:domain | string, integer | The domain name or id |
Add name servers for the domain
example.com
:
curl -v https://api.dynu.com/v1/domain/name_server_add/example.com \
-d "{\"name_servers\": [\"ns6.dynu.com\",\"ns7.dynu.com\"]}" \
-H "Content-Type: application/json" \
-H "Authorization: Bearer <token>"
Response-d "{\"name_servers\": [\"ns6.dynu.com\",\"ns7.dynu.com\"]}" \
-H "Content-Type: application/json" \
-H "Authorization: Bearer <token>"
Responds with HTTP/1.1 200 OK if successful, returns the name servers.
{
"name servers": [
"NS1.DYNU.COM",
"NS2.DYNU.COM",
"NS3.DYNU.COM",
"NS4.DYNU.COM",
"NS5.DYNU.COM"
"NS6.DYNU.COM"
"NS7.DYNU.COM"
]
}
"name servers": [
"NS1.DYNU.COM",
"NS2.DYNU.COM",
"NS3.DYNU.COM",
"NS4.DYNU.COM",
"NS5.DYNU.COM"
"NS6.DYNU.COM"
"NS7.DYNU.COM"
]
}
3
Delete name servers for a domain name
POST /v1/domain/name_server_remove/:domain
Parameters
Name | Type | Description |
---|---|---|
:domain | string, integer | The domain name or id |
Remove name servers for the domain
example.com
:
curl -v https://api.dynu.com/v1/domain/name_server_remove/example.com \
-d "{\"name_servers\": [\"ns6.dynu.com\",\"ns7.dynu.com\"]}" \
-H "Content-Type: application/json" \
-H "Authorization: Bearer <token>"
Response-d "{\"name_servers\": [\"ns6.dynu.com\",\"ns7.dynu.com\"]}" \
-H "Content-Type: application/json" \
-H "Authorization: Bearer <token>"
Responds with HTTP/1.1 200 OK if successful, returns the name servers.
{
"name servers": [
"NS1.DYNU.COM",
"NS2.DYNU.COM",
"NS3.DYNU.COM",
"NS4.DYNU.COM",
"NS5.DYNU.COM"
]
}
"name servers": [
"NS1.DYNU.COM",
"NS2.DYNU.COM",
"NS3.DYNU.COM",
"NS4.DYNU.COM",
"NS5.DYNU.COM"
]
}
4
Make a name server primary for a domain name
POST /v1/domain/name_server_primary/:domain
Parameters
Name | Type | Description |
---|---|---|
:domain | string, integer | The domain name or id |
Make
NS3.DYNU.COM
the primary name server for the domain example.com
:
curl -v https://api.dynu.com/v1/domain/name_server_primary/example.com \
-d "{\"name_servers\": \"ns3.dynu.com\"}" \
-H "Content-Type: application/json" \
-H "Authorization: Bearer <token>"
Response-d "{\"name_servers\": \"ns3.dynu.com\"}" \
-H "Content-Type: application/json" \
-H "Authorization: Bearer <token>"
Responds with HTTP/1.1 200 OK if successful, returns the name servers with the primary name server in the beginning.
{
"name servers": [
"NS3.DYNU.COM",
"NS1.DYNU.COM",
"NS2.DYNU.COM",
"NS4.DYNU.COM",
"NS5.DYNU.COM"
]
}
"name servers": [
"NS3.DYNU.COM",
"NS1.DYNU.COM",
"NS2.DYNU.COM",
"NS4.DYNU.COM",
"NS5.DYNU.COM"
]
}
DNS
Domains
1
Get a domain
GET /v1/dns/get/:domain
Parameters
Name | Type | Description |
---|---|---|
:domain | string | The domain name |
Get the domain
example.com
.
curl -v https://api.dynu.com/v1/dns/get/example.com \
-H "Content-Type: application/json" \
-H "Authorization: Bearer <token>"
Response-H "Content-Type: application/json" \
-H "Authorization: Bearer <token>"
Responds with HTTP/1.1 200 OK if successful, returns the domain.
{
"id": 1,
"user_id": 11,
"name": "example.com",
"token": "domain-token",
"state": "complete",
"ipv4_address":"174.23.124.56",
"ipv6_address": "2001:cdba::3257:9652",
"wildcard_alias": false,
"ttl": 1800,
"created_at": "2015-07-28T15:01:13",
"updated_at": "2015-09-10T13:03:15"
}
"id": 1,
"user_id": 11,
"name": "example.com",
"token": "domain-token",
"state": "complete",
"ipv4_address":"174.23.124.56",
"ipv6_address": "2001:cdba::3257:9652",
"wildcard_alias": false,
"ttl": 1800,
"created_at": "2015-07-28T15:01:13",
"updated_at": "2015-09-10T13:03:15"
}
2
List domains
GET /v1/dns/domains
Example
curl -v https://api.dynu.com/v1/dns/domains \
-H "Content-Type: application/json" \
-H "Authorization: Bearer <token>"
Response-H "Content-Type: application/json" \
-H "Authorization: Bearer <token>"
Responds with HTTP/1.1 200 OK if successful, returns a list of domain names.
[
{
"id": 1,
"user_id": 11,
"name": "example.com",
"token": "domain-token",
"state": "complete",
"ipv4_address":"174.23.124.56",
"ipv6_address": "fe80:0000:0000:0000:0202:b3ff:fe1e:8329",
"wildcard_alias": false,
"ttl": 1800,
"created_at": "2015-07-28T15:01:13",
"updated_at": "2015-09-10T13:03:15"
},
{
"id": 2,
"user_id": 11,
"name": "example.org",
"token": "domain-token",
"state": "complete",
"ipv4_address":"216.83.154.36",
"ipv6_address": "2001:0db8:0000:0000:0240:63ff:feca:9a21",
"wildcard_alias": false,
"ttl": 1800,
"created_at": "2015-08-18T05:01:13",
"updated_at": "2015-11-10T19:03:15"
},
{
"id": 3,
"user_id": 11,
"name": "example.net",
"token": "domain-token",
"state": "complete",
"ipv4_address":"184.101.179.7",
"ipv6_address": "2602:00ae:1a06:ce00:9d0e:3e91:89eb:d59f",
"wildcard_alias": false,
"ttl": 1800,
"created_at": "2015-09-10T15:10:56",
"updated_at": "2015-09-22T13:03:26"
}
]
{
"id": 1,
"user_id": 11,
"name": "example.com",
"token": "domain-token",
"state": "complete",
"ipv4_address":"174.23.124.56",
"ipv6_address": "fe80:0000:0000:0000:0202:b3ff:fe1e:8329",
"wildcard_alias": false,
"ttl": 1800,
"created_at": "2015-07-28T15:01:13",
"updated_at": "2015-09-10T13:03:15"
},
{
"id": 2,
"user_id": 11,
"name": "example.org",
"token": "domain-token",
"state": "complete",
"ipv4_address":"216.83.154.36",
"ipv6_address": "2001:0db8:0000:0000:0240:63ff:feca:9a21",
"wildcard_alias": false,
"ttl": 1800,
"created_at": "2015-08-18T05:01:13",
"updated_at": "2015-11-10T19:03:15"
},
{
"id": 3,
"user_id": 11,
"name": "example.net",
"token": "domain-token",
"state": "complete",
"ipv4_address":"184.101.179.7",
"ipv6_address": "2602:00ae:1a06:ce00:9d0e:3e91:89eb:d59f",
"wildcard_alias": false,
"ttl": 1800,
"created_at": "2015-09-10T15:10:56",
"updated_at": "2015-09-22T13:03:26"
}
]
3
Delete a domain
Delete the given domain from your account.
GET /v1/dns/delete/:domain
Parameters
Name | Type | Description |
---|---|---|
:domain | string | The domain name |
Delete the domain
example.com
.
curl -v https://api.dynu.com/v1/dns/delete/example.com \
-H "Content-Type: application/json" \
-H "Authorization: Bearer <token>"
Response-H "Content-Type: application/json" \
-H "Authorization: Bearer <token>"
Responds with HTTP/1.1 200 OK if successful.
4
Add DNS for a domain
POST /v1/dns/add/:domain
ExampleAdd DNS for domain name
example.com
with IP address 192.168.0.8 and IPv6 address 2001:cdba::3257:9652.
curl -v -X POST https://api.dynu.com/v1/dns/add \
-d "{\"name\": \"example.com\", \"ipv4_address\":\"192.168.0.8\", \"ipv6_address\":\"2001:cdba::3257:9652\"}" \
-H "Content-Type: application/json" \
-H "Authorization: Bearer <token>"
Response-d "{\"name\": \"example.com\", \"ipv4_address\":\"192.168.0.8\", \"ipv6_address\":\"2001:cdba::3257:9652\"}" \
-H "Content-Type: application/json" \
-H "Authorization: Bearer <token>"
Responds with HTTP/1.1 200 OK if successful, returns the domain.
{
"id": 1,
"user_id": 11,
"name": "example.com",
"token": "domain-token",
"state": "complete",
"ipv4_address":"192.168.0.8",
"ipv6_address": "2001:cdba::3257:9652",
"wildcard_alias": false,
"ttl": "1800",
"created_at": "2015-07-28T15:01:13",
"updated_at": "2015-09-10T13:03:15"
}
"id": 1,
"user_id": 11,
"name": "example.com",
"token": "domain-token",
"state": "complete",
"ipv4_address":"192.168.0.8",
"ipv6_address": "2001:cdba::3257:9652",
"wildcard_alias": false,
"ttl": "1800",
"created_at": "2015-07-28T15:01:13",
"updated_at": "2015-09-10T13:03:15"
}
IPv6 Address
1
Enable IPv6 address
GET /v1/dns/enableipv6/:domain
Parameters
Name | Type | Description |
---|---|---|
:domain | string, integer | The domain name or id |
Enable IPv6 address for
example.com
.
curl -v https://api.dynu.com/v1/dns/enableipv6/example.com \
-H "Content-Type: application/json" \
-H "Authorization: Bearer <token>"
Response-H "Content-Type: application/json" \
-H "Authorization: Bearer <token>"
Responds with HTTP/1.1 200 OK if successful, returns the domain.
{
"id": 1,
"user_id": 11,
"name": "example.com",
"token": "domain-token",
"state": "complete",
"ipv4_address":"174.23.124.56",
"ipv6_address": "2001:cdba::3257:9652",
"wildcard_alias": true,
"ttl": 1800,
"created_at": "2015-07-28T15:01:13",
"updated_at": "2015-09-10T13:03:15"
}
"id": 1,
"user_id": 11,
"name": "example.com",
"token": "domain-token",
"state": "complete",
"ipv4_address":"174.23.124.56",
"ipv6_address": "2001:cdba::3257:9652",
"wildcard_alias": true,
"ttl": 1800,
"created_at": "2015-07-28T15:01:13",
"updated_at": "2015-09-10T13:03:15"
}
2
Disable IPv6 address
GET /v1/dns/disableipv6/:domain
Parameters
Name | Type | Description |
---|---|---|
:domain | string, integer | The domain name or id |
Disable IPv6 address for
example.com
.
curl -v https://api.dynu.com/v1/dns/disableipv6/example.com \
-H "Content-Type: application/json" \
-H "Authorization: Bearer <token>"
Response-H "Content-Type: application/json" \
-H "Authorization: Bearer <token>"
Responds with HTTP/1.1 200 OK if successful, returns the domain.
{
"id": 1,
"user_id": 11,
"name": "example.com",
"token": "domain-token",
"state": "complete",
"ipv4_address":"174.23.124.56",
"ipv6_address": "",
"wildcard_alias": false,
"ttl": 1800,
"created_at": "2015-07-28T15:01:13",
"updated_at": "2015-09-10T13:03:15"
}
"id": 1,
"user_id": 11,
"name": "example.com",
"token": "domain-token",
"state": "complete",
"ipv4_address":"174.23.124.56",
"ipv6_address": "",
"wildcard_alias": false,
"ttl": 1800,
"created_at": "2015-07-28T15:01:13",
"updated_at": "2015-09-10T13:03:15"
}
Wildcard Alias
1
Enable IPv4/IPv6 wildcard alias
GET /v1/dns/enableipv4wildcard/:domain
GET /v1/dns/enableipv6wildcard/:domain
Parameters
GET /v1/dns/enableipv6wildcard/:domain
Name | Type | Description |
---|---|---|
:domain | string | The domain name |
Enable IPv4 wildcard alias for
example.com
.
curl -v https://api.dynu.com/v1/dns/enableipv4wildcard/example.com \
-H "Content-Type: application/json" \
-H "Authorization: Bearer <token>"
Response-H "Content-Type: application/json" \
-H "Authorization: Bearer <token>"
Responds with HTTP/1.1 200 OK if successful, returns the domain.
{
"id": 1,
"user_id": 11,
"name": "example.com",
"token": "EKQKSSRPSFTXUPNLYIEZ",
"state": "complete",
"ipv4_address":"174.23.124.56",
"ipv6_address": "",
"wildcard_alias": true,
"ttl": 1800,
"created_at": "2015-07-28T15:01:13",
"updated_at": "2015-09-10T13:03:15"
}
"id": 1,
"user_id": 11,
"name": "example.com",
"token": "EKQKSSRPSFTXUPNLYIEZ",
"state": "complete",
"ipv4_address":"174.23.124.56",
"ipv6_address": "",
"wildcard_alias": true,
"ttl": 1800,
"created_at": "2015-07-28T15:01:13",
"updated_at": "2015-09-10T13:03:15"
}
2
Disable IPv4/IPv6 wildcard alias
GET /v1/dns/disableipv4wildcard/:domain
GET /v1/dns/disableipv6wildcard/:domain
Parameters
GET /v1/dns/disableipv6wildcard/:domain
Name | Type | Description |
---|---|---|
:domain | string | The domain name |
Disable IPv4 wildcard alias for
example.com
.
curl -v https://api.dynu.com/v1/dns/disableipv4wildcard/example.com \
-H "Content-Type: application/json" \
-H "Authorization: Bearer <token>"
Response-H "Content-Type: application/json" \
-H "Authorization: Bearer <token>"
Responds with HTTP/1.1 200 OK if successful, returns the domain.
{
"id": 1,
"user_id": 11,
"name": "example.com",
"token": "EKQKSSRPSFTXUPNLYIEZ",
"state": "complete",
"ipv4_address":"174.23.124.56",
"ipv6_address": "",
"wildcard_alias": false,
"ttl": 1800,
"created_at": "2015-07-28T15:01:13",
"updated_at": "2015-09-10T13:03:15"
}
"id": 1,
"user_id": 11,
"name": "example.com",
"token": "EKQKSSRPSFTXUPNLYIEZ",
"state": "complete",
"ipv4_address":"174.23.124.56",
"ipv6_address": "",
"wildcard_alias": false,
"ttl": 1800,
"created_at": "2015-07-28T15:01:13",
"updated_at": "2015-09-10T13:03:15"
}
DNS Records
1
List records for a domain name
GET /v1/dns/records/:domain
Parameters
Name | Type | Description |
---|---|---|
:domain | string | The domain name |
List records for domain
example.com
.
curl -v https://api.dynu.com/v1/dns/records/example.com \
-H "Content-Type: application/json" \
-H "Authorization: Bearer <token>"
Response-H "Content-Type: application/json" \
-H "Authorization: Bearer <token>"
Responds with HTTP/1.1 200 OK if successful, returns the list of DNS records
[
{
"host": "mx1.dynu.com",
"priority": 10,
"id": 1852562,
"domain_id": 7341211,
"domain_name": "example.com",
"node_name": "",
"hostname": "example.com",
"record_type": "MX",
"ttl": 1800,
"content": "10 mx1.dynu.com",
"state": true
},
{
"host": "mx2.dynu.com",
"priority": 20,
"id": 1852563,
"domain_id": 7341211,
"domain_name": "example.com",
"node_name": "",
"hostname": "example.com",
"record_type": "MX",
"ttl": 1800,
"content": "20 mx2.dynu.com",
"state": true
},
{
"host": "somedomain.com",
"id": 1852588,
"domain_id": 7341211,
"domain_name": "example.com",
"node_name": "mail",
"hostname": "mail.example.com",
"record_type": "CNAME",
"ttl": 1800,
"content": "somedomain.com",
"state": true
},
{
"text_data": "This is some txt record.",
"id": 1852589,
"domain_id": 7341211,
"domain_name": "example.com",
"node_name": "",
"hostname": "example.com",
"record_type": "TXT",
"ttl": 1800,
"content": "This is some txt record.",
"state": false
}
]
{
"host": "mx1.dynu.com",
"priority": 10,
"id": 1852562,
"domain_id": 7341211,
"domain_name": "example.com",
"node_name": "",
"hostname": "example.com",
"record_type": "MX",
"ttl": 1800,
"content": "10 mx1.dynu.com",
"state": true
},
{
"host": "mx2.dynu.com",
"priority": 20,
"id": 1852563,
"domain_id": 7341211,
"domain_name": "example.com",
"node_name": "",
"hostname": "example.com",
"record_type": "MX",
"ttl": 1800,
"content": "20 mx2.dynu.com",
"state": true
},
{
"host": "somedomain.com",
"id": 1852588,
"domain_id": 7341211,
"domain_name": "example.com",
"node_name": "mail",
"hostname": "mail.example.com",
"record_type": "CNAME",
"ttl": 1800,
"content": "somedomain.com",
"state": true
},
{
"text_data": "This is some txt record.",
"id": 1852589,
"domain_id": 7341211,
"domain_name": "example.com",
"node_name": "",
"hostname": "example.com",
"record_type": "TXT",
"ttl": 1800,
"content": "This is some txt record.",
"state": false
}
]
2
Create a record
POST /v1/dns/record/add
Base Input
Name | Type | Description |
---|---|---|
domain_name | string | Required. |
node_name | string | Optional. Default is empty. Required for CNAME and RP record. |
record_type | string | Required. A, AAAA, CANME, TXT etc. |
ttl | integer | Optional. Default is 90 seconds. |
state | bool | Required. |
A record
Input
Name | Type | Description |
---|---|---|
location | string | For A and AAAA record. Optional. Default is empty. Used for IP update. |
ipv4_address | string | For A record. Optional. Default is the IP address from where the request originates. |
Add an A record for domain name
example.com
with node namesub
.
curl -v -X POST https://api.dynu.com/v1/dns/record/add \
-d "{\"ipv4_address\":\"192.168.0.1\", \"ipv6_address\":\"2001:0db8:85a3:0000:0000:8a2e:0370:7334\", \"domain_name\":\"example.com\", \"node_name\":\"sub\", \"record_type\":\"A\", \"ttl\":\"1800\", \"state\":\"true\" }" \
-H "Content-Type: application/json" \
-H "Authorization: Bearer <token>"
Response-d "{\"ipv4_address\":\"192.168.0.1\", \"ipv6_address\":\"2001:0db8:85a3:0000:0000:8a2e:0370:7334\", \"domain_name\":\"example.com\", \"node_name\":\"sub\", \"record_type\":\"A\", \"ttl\":\"1800\", \"state\":\"true\" }" \
-H "Content-Type: application/json" \
-H "Authorization: Bearer <token>"
Responds with HTTP/1.1 200 OK if successful, returns the DNS record.
{
"location": "",
"ipv4_address": "192.168.0.1",
"id": 1274272,
"domain_id": 7341211,
"domain_name": "example.com",
"node_name": "sub",
"hostname": "sub.example.com",
"record_type": "A",
"ttl": 1800,
"content": "192.168.0.1",
"state": true
}
Responds with with the error message if bad request.
"location": "",
"ipv4_address": "192.168.0.1",
"id": 1274272,
"domain_id": 7341211,
"domain_name": "example.com",
"node_name": "sub",
"hostname": "sub.example.com",
"record_type": "A",
"ttl": 1800,
"content": "192.168.0.1",
"state": true
}
AAAA record
Input
Name | Type | Description |
---|---|---|
location | string | For A and AAAA record. Optional. Default is empty. Used for IP update. |
ipv6_address | string | For AAAA record. Optional. Default is the IP address from where the request originates. |
Add an AAAA record for domain name
example.com
with node namesub
.
curl -v -X POST https://api.dynu.com/v1/dns/record/add \
-d "{\"ipv6_address\":\"2002:4559:1FE2:0:0:0:4559:1FE2\", \"domain_name\":\"example.com\", \"node_name\":\"sub\", \"record_type\":\"AAAA\", \"ttl\":\"1800\", \"state\":\"true\" }" \
-H "Content-Type: application/json" \
-H "Authorization: Bearer <token>"
Response-d "{\"ipv6_address\":\"2002:4559:1FE2:0:0:0:4559:1FE2\", \"domain_name\":\"example.com\", \"node_name\":\"sub\", \"record_type\":\"AAAA\", \"ttl\":\"1800\", \"state\":\"true\" }" \
-H "Content-Type: application/json" \
-H "Authorization: Bearer <token>"
Responds with HTTP/1.1 200 OK if successful, returns the DNS record.
{
"location": "",
"ipv6_address": "2002:4559:1FE2:0:0:0:4559:1FE2",
"id": 1274273,
"domain_id": 7341211,
"domain_name": "example.com",
"node_name": "sub",
"hostname": "sub.example.com",
"record_type": "AAAA",
"ttl": 1800,
"content": "2002:4559:1FE2:0:0:0:4559:1FE2",
"state": true
}
Responds with with the error message if bad request.
"location": "",
"ipv6_address": "2002:4559:1FE2:0:0:0:4559:1FE2",
"id": 1274273,
"domain_id": 7341211,
"domain_name": "example.com",
"node_name": "sub",
"hostname": "sub.example.com",
"record_type": "AAAA",
"ttl": 1800,
"content": "2002:4559:1FE2:0:0:0:4559:1FE2",
"state": true
}
AFSDB record
Input
Name | Type | Description |
---|---|---|
host | string | For AFSDB, CNAME, MX record. Required. |
sub_type | int | For AFSDB record. Required. AFSDBSubType(enum):1,2,3. |
Add an AFSDB record for domain name
example.com
.
curl -v -X POST https://api.dynu.com/v1/dns/record/add \
-d "{\"host\":\"myserver.com\", \"sub_type\":\"1\", \"domain_name\":\"example.com\", \"node_name\":\"\", \"record_type\":\"AFSDB\", \"ttl\":\"1800\", \"state\":\"false\" }" \
-H "Content-Type: application/json" \
-H "Authorization: Bearer <token>"
Response-d "{\"host\":\"myserver.com\", \"sub_type\":\"1\", \"domain_name\":\"example.com\", \"node_name\":\"\", \"record_type\":\"AFSDB\", \"ttl\":\"1800\", \"state\":\"false\" }" \
-H "Content-Type: application/json" \
-H "Authorization: Bearer <token>"
Responds with HTTP/1.1 200 OK if successful, returns the DNS record.
{
"host": "myserver.com",
"sub_type": "1",
"id": 1274274,
"domain_id": 7341211,
"domain_name": "example.com",
"node_name": "",
"hostname": "example.com",
"record_type": "AFSDB",
"ttl": 1800,
"content": "1 myserver.com",
"state": false
}
Responds with with the error message if bad request.
"host": "myserver.com",
"sub_type": "1",
"id": 1274274,
"domain_id": 7341211,
"domain_name": "example.com",
"node_name": "",
"hostname": "example.com",
"record_type": "AFSDB",
"ttl": 1800,
"content": "1 myserver.com",
"state": false
}
HINFO record
Input
Name | Type | Description |
---|---|---|
hardware | string | For HINFO record. Required. |
operating_system | string | For HINFO record. Required. |
Add an HINFO for domain name
example.com
.
curl -v -X POST https://api.dynu.com/v1/dns/record/add \
-d "{\"hardware\":\"Intel Xeon\", \"operating_system\":\"Windows\", \"record_type\":\"HINFO\", \"ttl\":\"1800\", \"state\":\"true\" }" \
-H "Content-Type: application/json" \
-H "Authorization: Bearer <token>"
Response-d "{\"hardware\":\"Intel Xeon\", \"operating_system\":\"Windows\", \"record_type\":\"HINFO\", \"ttl\":\"1800\", \"state\":\"true\" }" \
-H "Content-Type: application/json" \
-H "Authorization: Bearer <token>"
Responds with HTTP/1.1 200 OK if successful, returns the DNS record.
{
"hardware": "Intel Xeon",
"operating_system": "Windows",
"id": 1274276,
"domain_id": 7341211,
"domain_name": "example.com",
"node_name": "",
"hostname": "example.com",
"record_type": "HINFO",
"ttl": 1800,
"content": "Intel Xeon Windows",
"state": true
}
Responds with with the error message if bad request.
"hardware": "Intel Xeon",
"operating_system": "Windows",
"id": 1274276,
"domain_id": 7341211,
"domain_name": "example.com",
"node_name": "",
"hostname": "example.com",
"record_type": "HINFO",
"ttl": 1800,
"content": "Intel Xeon Windows",
"state": true
}
Key record
Input
Name | Type | Description |
---|---|---|
flags | string | For Key record. Required. 16 characters, e.g."0100000100000001". |
protocol | string | For Key record. Required. KEYProtocol(enum), {TLS, Email, DNSSEC, IPSEC, All}. |
algorithm | string | For Key record. Required. KEYAlgorithm(enum:value), {RSAMD5, DiffieHellman, DSA, EllipticCurveCryptography, RSASHA1, IndirectKey}. |
key | string | For Key record. Required. |
Add a KEY record for domain name
example.com
.
curl -v -X POST https://api.dynu.com/v1/dns/record/add \
-d "{\"flags\":\"0100000100000101\", \"protocol\":\"Email\", \"algorithm\":\"RSAMD5\", \"key\":\"SomeBase64encodedstuff\", \"domain_name\":\"example.com\", \"record_type\":\"KEY\", \"ttl\":\"1800\", \"state\":\"true\" }" \
-H "Content-Type: application/json" \
-H "Authorization: Bearer <token>"
Response-d "{\"flags\":\"0100000100000101\", \"protocol\":\"Email\", \"algorithm\":\"RSAMD5\", \"key\":\"SomeBase64encodedstuff\", \"domain_name\":\"example.com\", \"record_type\":\"KEY\", \"ttl\":\"1800\", \"state\":\"true\" }" \
-H "Content-Type: application/json" \
-H "Authorization: Bearer <token>"
Responds with HTTP/1.1 200 OK if successful, returns the DNS record.
{
"flags": "0100000100000001",
"protocol": "2",
"algorithm": "1",
"key": "SomeBase64encodedstuff",
"id": 1855570,
"domain_id": 7341211,
"domain_name": "example.com",
"node_name": "",
"hostname": "example.com",
"record_type": "KEY",
"ttl": 1800,
"content": "0100000100000101 2 1 SomeBase64encodedstuff",
"state": true
}
Responds with with the error message if bad request.
"flags": "0100000100000001",
"protocol": "2",
"algorithm": "1",
"key": "SomeBase64encodedstuff",
"id": 1855570,
"domain_id": 7341211,
"domain_name": "example.com",
"node_name": "",
"hostname": "example.com",
"record_type": "KEY",
"ttl": 1800,
"content": "0100000100000101 2 1 SomeBase64encodedstuff",
"state": true
}
MX record
Input
Name | Type | Description |
---|---|---|
host | string | For AFSDB, CNAME, MX record. Required. |
priority | int | For TXT, SPF record. Optional. Default is 10. |
Add an MX record for domain name
example.com
.
curl -v -X POST https://api.dynu.com/v1/dns/record/add \
-d "{\"host\":\"mx2.dynu.com\", \"priority\":\"20\", \"domain_name\":\"example.com\", \"record_type\":\"MX\", \"ttl\":\"1800\", \"state\":\"true\" }" \
-H "Content-Type: application/json" \
-H "Authorization: Bearer <token>"
Response-d "{\"host\":\"mx2.dynu.com\", \"priority\":\"20\", \"domain_name\":\"example.com\", \"record_type\":\"MX\", \"ttl\":\"1800\", \"state\":\"true\" }" \
-H "Content-Type: application/json" \
-H "Authorization: Bearer <token>"
Responds with HTTP/1.1 200 OK if successful, returns the DNS record.
{
"host": "mx2.dynu.com",
"priority": "20",
"id": 1274282,
"domain_id": 7341211,
"domain_name": "example.com",
"node_name": "",
"hostname": "example.com",
"record_type": "MX",
"ttl": 1800,
"content": "20 mx2.dynu.com",
"state": true
}
Responds with with the error message if bad request.
"host": "mx2.dynu.com",
"priority": "20",
"id": 1274282,
"domain_id": 7341211,
"domain_name": "example.com",
"node_name": "",
"hostname": "example.com",
"record_type": "MX",
"ttl": 1800,
"content": "20 mx2.dynu.com",
"state": true
}
NS record
Input
Name | Type | Description |
---|---|---|
name_server | string | For NS record. Required. |
Add an NS record for domain name
example.com
.
curl -v -X POST https://api.dynu.com/v1/dns/record/add \
-d "{\"name_server\":\"ns9.dynu.com\", \"domain_name\":\"example.com\", \"record_type\":\"NS\", \"ttl\":\"1800\", \"state\":\"true\" }" \
-H "Content-Type: application/json" \
-H "Authorization: Bearer <token>"
Response-d "{\"name_server\":\"ns9.dynu.com\", \"domain_name\":\"example.com\", \"record_type\":\"NS\", \"ttl\":\"1800\", \"state\":\"true\" }" \
-H "Content-Type: application/json" \
-H "Authorization: Bearer <token>"
Responds with HTTP/1.1 200 OK if successful, returns the DNS record.
{
"name_server": "ns9.dynu.com",
"id": 1855539,
"domain_id": 7341211,
"domain_name": "example.com",
"node_name": "",
"hostname": "example.com",
"record_type": "NS",
"ttl": 1800,
"content": "ns9.dynu.com",
"state": true
}
Responds with with the error message if bad request.
"name_server": "ns9.dynu.com",
"id": 1855539,
"domain_id": 7341211,
"domain_name": "example.com",
"node_name": "",
"hostname": "example.com",
"record_type": "NS",
"ttl": 1800,
"content": "ns9.dynu.com",
"state": true
}
PF record
Input
Name | Type | Description |
---|---|---|
host | string | For PF record. Optional. Default is empty. |
port | int | For PF record. Optional. Default is 81. |
title | string | For PF record. Optional. Default is empty. |
meta_keywords | string | For PF record. Optional. Default is empty. |
meta_description | string | For PF record. Optional. Default is empty. |
cloak | bool | For PF record. Optional. Default is false. |
include_query_string | bool | For PF record. Optional. Default is true. |
use_dynamic_ipv4_address | bool | For PF record. Optional. Default is true. |
use_dynamic_ipv6_address | bool | For PF record. Optional. Default is true. |
Add a port forwarding record for hostname name
blog.example.com
to redirect to redirectpage.com
on port 8080.
curl -v -X POST https://api.dynu.com/v1/dns/record/add \
-d "{\"host\":\"redirectpage.com\", \"port\":\"8080\", \"title\":\"My home page\", \"meta_keywords\":\"photo, travel, island, beach\", \"meta_description\":\"This is my personal blog site with travel logs.\", \"cloak\":\"true\", \"include_query_string\":\"true\", \"use_dynamic_ipv4_address\":\"true\", \"use_dynamic_ipv6_address\":\"false\", \"domain_name\":\"example.com\", \"node_name\":\"blog\", \"record_type\":\"PF\" \"state\":\"true\" }" \
-H "Content-Type: application/json" \
-H "Authorization: Bearer <token>"
Response-d "{\"host\":\"redirectpage.com\", \"port\":\"8080\", \"title\":\"My home page\", \"meta_keywords\":\"photo, travel, island, beach\", \"meta_description\":\"This is my personal blog site with travel logs.\", \"cloak\":\"true\", \"include_query_string\":\"true\", \"use_dynamic_ipv4_address\":\"true\", \"use_dynamic_ipv6_address\":\"false\", \"domain_name\":\"example.com\", \"node_name\":\"blog\", \"record_type\":\"PF\" \"state\":\"true\" }" \
-H "Content-Type: application/json" \
-H "Authorization: Bearer <token>"
Responds with HTTP/1.1 200 OK if successful, returns the DNS record.
{
"host": "redirectpage.com",
"port": "8080",
"redirect_url": http://redirectpage.com:8080"",
"title": "My home page",
"meta_keywords": "photo, travel, island, beach",
"meta_description": "This is my personal blog site with travel logs.",
"cloak": "true",
"include_query_string": "true",
"use_dynamic_ipv4_address": "true",
"use_dynamic_ipv6_address": "false",
"id": 1855542,
"domain_id": 7341211,
"domain_name": "example.com",
"node_name": "blog",
"hostname": "blog.example.com",
"record_type": "PF",
"ttl": 90,
"content": "http://redirectpage.com:8080",
"state": true
}
Responds with with the error message if bad request..
"host": "redirectpage.com",
"port": "8080",
"redirect_url": http://redirectpage.com:8080"",
"title": "My home page",
"meta_keywords": "photo, travel, island, beach",
"meta_description": "This is my personal blog site with travel logs.",
"cloak": "true",
"include_query_string": "true",
"use_dynamic_ipv4_address": "true",
"use_dynamic_ipv6_address": "false",
"id": 1855542,
"domain_id": 7341211,
"domain_name": "example.com",
"node_name": "blog",
"hostname": "blog.example.com",
"record_type": "PF",
"ttl": 90,
"content": "http://redirectpage.com:8080",
"state": true
}
PTR record
Input
Name | Type | Description |
---|---|---|
host | string | For PTR record. Required. |
Add a PTR for hostname
testptr.example.com
.
curl -v -X POST https://api.dynu.com/v1/dns/record/add \
-d "{\"host\":\"somedomain.com\", \"domain_name\":\"example.com\", \"node_name\":\"testptr\", \"record_type\":\"PTR\", \"ttl\":\"1800\", \"state\":\"true\" }" \
-H "Content-Type: application/json" \
-H "Authorization: Bearer <token>"
Response-d "{\"host\":\"somedomain.com\", \"domain_name\":\"example.com\", \"node_name\":\"testptr\", \"record_type\":\"PTR\", \"ttl\":\"1800\", \"state\":\"true\" }" \
-H "Content-Type: application/json" \
-H "Authorization: Bearer <token>"
Responds with HTTP/1.1 200 OK if successful, returns the DNS record.
{
"host": "somedomain.com",
"id": 1855555,
"domain_id": 7341211,
"domain_name": "example.com",
"node_name": "testptr",
"hostname": "testptr.example.com",
"record_type": "PTR",
"ttl": 1800,
"content": "somedomain.com",
"state": true
}
Responds with with the error message if bad request.
"host": "somedomain.com",
"id": 1855555,
"domain_id": 7341211,
"domain_name": "example.com",
"node_name": "testptr",
"hostname": "testptr.example.com",
"record_type": "PTR",
"ttl": 1800,
"content": "somedomain.com",
"state": true
}
RP record
Input
Name | Type | Description |
---|---|---|
mailbox | string | For RP record. Required. |
txt_domain_name | string | For RP record. Required. |
Add an RP record for hostname
testrp.example.com
.
curl -v -X POST https://api.dynu.com/v1/dns/record/add \
-d "{\"mailbox\":\"admin.example.com\", \"txt_domain_name\":\"Phone: (800)-456-890\", \"domain_name\":\"example.com\", \"node_name\":\"testtp\", \"record_type\":\"AAAA\", \"ttl\":\"1800\", \"state\":\"true\" }" \
-H "Content-Type: application/json" \
-H "Authorization: Bearer <token>"
Response-d "{\"mailbox\":\"admin.example.com\", \"txt_domain_name\":\"Phone: (800)-456-890\", \"domain_name\":\"example.com\", \"node_name\":\"testtp\", \"record_type\":\"AAAA\", \"ttl\":\"1800\", \"state\":\"true\" }" \
-H "Content-Type: application/json" \
-H "Authorization: Bearer <token>"
Responds with HTTP/1.1 200 OK if successful, returns the DNS record.
{
"mailbox": "admin.example.com",
"txt_domain_name": "Phone: (800)-456-890",
"id": 1855559,
"domain_id": 7341211,
"domain_name": "example.com",
"node_name": "testrp",
"hostname": "testrp.example.com",
"record_type": "RP",
"ttl": 1800,
"content": "admin.example.com Phone: (800)-456-890",
"state": true
}
Responds with with the error message if bad request.
"mailbox": "admin.example.com",
"txt_domain_name": "Phone: (800)-456-890",
"id": 1855559,
"domain_id": 7341211,
"domain_name": "example.com",
"node_name": "testrp",
"hostname": "testrp.example.com",
"record_type": "RP",
"ttl": 1800,
"content": "admin.example.com Phone: (800)-456-890",
"state": true
}
SPF record
Input
Name | Type | Description |
---|---|---|
text_data | string | For TXT, SPF record. Required |
Add an SPF record for domain name
example.com
.
curl -v -X POST https://api.dynu.com/v1/dns/record/add \
-d "{\"text_data\":\"v=spf1 a include:_spf.google.com ~all\", \"domain_name\":\"example.com\", \"record_type\":\"SPF\", \"ttl\":\"1800\", \"state\":\"true\" }" \
-H "Content-Type: application/json" \
-H "Authorization: Bearer <token>"
Response-d "{\"text_data\":\"v=spf1 a include:_spf.google.com ~all\", \"domain_name\":\"example.com\", \"record_type\":\"SPF\", \"ttl\":\"1800\", \"state\":\"true\" }" \
-H "Content-Type: application/json" \
-H "Authorization: Bearer <token>"
Responds with HTTP/1.1 200 OK if successful, returns the DNS record.
{
"text_data": "v=spf1 a include:_spf.google.com ~all",
"id": 1855563,
"domain_id": 7341211,
"domain_name": "example.com",
"node_name": "",
"hostname": "example.com",
"record_type": "SPF",
"ttl": 1800,
"content": "v=spf1 a include:_spf.google.com ~all",
"state": true
}
Responds with with the error message if bad request.
"text_data": "v=spf1 a include:_spf.google.com ~all",
"id": 1855563,
"domain_id": 7341211,
"domain_name": "example.com",
"node_name": "",
"hostname": "example.com",
"record_type": "SPF",
"ttl": 1800,
"content": "v=spf1 a include:_spf.google.com ~all",
"state": true
}
TXT record
Input
Name | Type | Description |
---|---|---|
text_data | string | For TXT, SPF record. Required |
Add a txt record for domain name
example.com
with node nametesttxt
.
curl -v -X POST https://api.dynu.com/v1/dns/record/add \
-d "{\"text_data\":\"This is a test TXT message.\", \"domain_name\":\"example.com\", \"node_name\":\"testtxt\", \"record_type\":\"TXT\", \"ttl\":\"1800\", \"state\":\"true\" }" \
-H "Content-Type: application/json" \
-H "Authorization: Bearer <token>"
Response-d "{\"text_data\":\"This is a test TXT message.\", \"domain_name\":\"example.com\", \"node_name\":\"testtxt\", \"record_type\":\"TXT\", \"ttl\":\"1800\", \"state\":\"true\" }" \
-H "Content-Type: application/json" \
-H "Authorization: Bearer <token>"
Responds with HTTP/1.1 200 OK if successful, returns the DNS record.
{
"text_data": "This is a test TXT message.",
"id": 1855563,
"domain_id": 7341211,
"domain_name": "example.com",
"node_name": "testtxt",
"hostname": "testtxt.example.com",
"record_type": "TXT",
"ttl": 1800,
"content": "This is a test TXT message",
"state": true
}
Responds with with the error message if bad request.
"text_data": "This is a test TXT message.",
"id": 1855563,
"domain_id": 7341211,
"domain_name": "example.com",
"node_name": "testtxt",
"hostname": "testtxt.example.com",
"record_type": "TXT",
"ttl": 1800,
"content": "This is a test TXT message",
"state": true
}
SRV record
Input
Name | Type | Description |
---|---|---|
port | int | For SRV record. Required |
priority | int | For SRV record. Required |
weight | int | For SRV record. Required |
target | string | For SRV record. Required |
service | string | For SRV record. Required |
protocol | string | For SRV record. Required |
Add an SRV record for hostname
minecraft.example.com
.
curl -v -X POST https://api.dynu.com/v1/dns/record/add \
-d "{\"port\":\"27165\", \"priority\":\"0\", \"weight\":\"5\", \"target\":\"myminecraft.com\", \"service\":\"minecraft\", \"protocol\":\"tcp\", \"domain_name\":\"example.com\", \"node_name\":\"minecraft\", \"record_type\":\"SRV\", \"ttl\":\"1800\", \"state\":\"true\" }" \
-H "Content-Type: application/json" \
-H "Authorization: Bearer <token>"
Response-d "{\"port\":\"27165\", \"priority\":\"0\", \"weight\":\"5\", \"target\":\"myminecraft.com\", \"service\":\"minecraft\", \"protocol\":\"tcp\", \"domain_name\":\"example.com\", \"node_name\":\"minecraft\", \"record_type\":\"SRV\", \"ttl\":\"1800\", \"state\":\"true\" }" \
-H "Content-Type: application/json" \
-H "Authorization: Bearer <token>"
Responds with HTTP/1.1 200 OK if successful, returns the DNS record.
{
"port": 27165,
"priority": "0",
"weight": "5",
"target": "myminecraft.com",
"service": "minecraft",
"protocol": "tcp",
"id": 1852848,
"domain_id": 7341211,
"domain_name": "example.com",
"node_name": "minecraft",
"hostname": "_minecraft._tcp._minecraft.minecraft.example.com",
"record_type": "SRV",
"ttl": 1800,
"content": "0 5 27165 myminecraft.com",
"state": true
Responds with with the error message if bad request.
"port": 27165,
"priority": "0",
"weight": "5",
"target": "myminecraft.com",
"service": "minecraft",
"protocol": "tcp",
"id": 1852848,
"domain_id": 7341211,
"domain_name": "example.com",
"node_name": "minecraft",
"hostname": "_minecraft._tcp._minecraft.minecraft.example.com",
"record_type": "SRV",
"ttl": 1800,
"content": "0 5 27165 myminecraft.com",
"state": true
UF record
Input
Name | Type | Description |
---|---|---|
redirect_url | string | For UF record. Required. For example, https://www.cnn.com. |
title | string | For PF record. Optional. Default is empty. |
meta_keywords | string | For PF record. Optional. Default is empty. |
meta_description | string | For PF record. Optional. Default is empty. |
cloak | bool | For PF record. Optional. Default is false. |
include_query_string | bool | For PF record. Optional. Default is true. |
Add a URL forwarding record for hostname
testuf.example.com
to forward to https://google.com.
curl -v -X POST https://api.dynu.com/v1/dns/record/add \
-d "{\"redirect_url\":\"https://google.com\", \"domain_name\":\"example.com\", \"node_name\":\"testuf\", \"record_type\":\"UF\", \"ttl\":\"1800\", \"state\":\"true\" }" \
-H "Content-Type: application/json" \
-H "Authorization: Bearer <token>"
Response-d "{\"redirect_url\":\"https://google.com\", \"domain_name\":\"example.com\", \"node_name\":\"testuf\", \"record_type\":\"UF\", \"ttl\":\"1800\", \"state\":\"true\" }" \
-H "Content-Type: application/json" \
-H "Authorization: Bearer <token>"
Responds with HTTP/1.1 200 OK if successful, returns the DNS record.
{
"redirect_url": "https://www.yahoo.com",
"title": "",
"meta_keywords": "",
"meta_description": "",
"cloak": "false",
"include_query_string": "false",
"id": 1855565,
"domain_id": 7341211,
"domain_name": "example.com",
"node_name": "testuf",
"hostname": "testuf.example.com",
"record_type": "UF",
"ttl": 1800,
"content": "https://google.com",
"state": true
}
Responds with with the error message if bad request.
"redirect_url": "https://www.yahoo.com",
"title": "",
"meta_keywords": "",
"meta_description": "",
"cloak": "false",
"include_query_string": "false",
"id": 1855565,
"domain_id": 7341211,
"domain_name": "example.com",
"node_name": "testuf",
"hostname": "testuf.example.com",
"record_type": "UF",
"ttl": 1800,
"content": "https://google.com",
"state": true
}
3
Get a record
GET /v1/dns/record/get/:id
Parameters
Name | Type | Description |
---|---|---|
:id | integer | The record id |
Get the record with ID
1852848
which is an SRV record for minecraft.example.com
:
curl -v https://api.dynu.com/v1/dns/record/get/1852848 \
-H "Content-Type: application/json" \
-H "Authorization: Bearer <token>"
Response-H "Content-Type: application/json" \
-H "Authorization: Bearer <token>"
Responds with HTTP/1.1 200 OK if successful, returns the DNS record.
{
"port": 27165,
"priority": "0",
"weight": "5",
"target": "myminecraft.com",
"service": "minecraft",
"protocol": "tcp",
"id": 1852848,
"domain_id": 7341211,
"domain_name": "example.com",
"node_name": "minecraft",
"hostname": "_minecraft._tcp._minecraft.minecraft.example.com",
"record_type": "SRV",
"ttl": 1800,
"content": "0 5 27165 myminecraft.com",
"state": true
}
"port": 27165,
"priority": "0",
"weight": "5",
"target": "myminecraft.com",
"service": "minecraft",
"protocol": "tcp",
"id": 1852848,
"domain_id": 7341211,
"domain_name": "example.com",
"node_name": "minecraft",
"hostname": "_minecraft._tcp._minecraft.minecraft.example.com",
"record_type": "SRV",
"ttl": 1800,
"content": "0 5 27165 myminecraft.com",
"state": true
}
4
Enable a record
GET /v1/dns/record/enable/:id
Parameters
Name | Type | Description |
---|---|---|
:id | integer | The record id |
Enable the record with ID
1852589
which is a TXT record for domain name example.com
.
curl -v https://api.dynu.com/v1/dns/record/enable/1852589 \
-H "Content-Type: application/json" \
-H "Authorization: Bearer <token>"
Response-H "Content-Type: application/json" \
-H "Authorization: Bearer <token>"
Returns the record with the state true.
{
"text_data": "This is some txt record.",
"id": 1852589,
"domain_id": 7341211,
"domain_name": "example.com",
"node_name": "",
"hostname": "example.com",
"record_type": "TXT",
"ttl": 1800,
"content": "This is some txt record.",
"state": true
}
"text_data": "This is some txt record.",
"id": 1852589,
"domain_id": 7341211,
"domain_name": "example.com",
"node_name": "",
"hostname": "example.com",
"record_type": "TXT",
"ttl": 1800,
"content": "This is some txt record.",
"state": true
}
5
Disable a record
GET /v1/dns/record/disable/:id
Parameters
Name | Type | Description |
---|---|---|
:id | integer | The record id |
Disable the record with ID
1852891
which is a RP (Responsible Person) record rp.example.com
.
curl -H 'X-Dynu-Token: <email>:<token>' \
-H 'Accept: application/json'\
-H 'Content-Type: application/json' \
https://api.dynu.com/v1/dns/record/disable/1852891
Response-H 'Accept: application/json'\
-H 'Content-Type: application/json' \
https://api.dynu.com/v1/dns/record/disable/1852891
Returns the record with the state false.
{
"mailbox": "admin.example.com",
"id": 1852891,
"domain_id": 7341211,
"domain_name": "example.com",
"node_name": "rp",
"hostname": "rp.example.com",
"record_type": "RP",
"ttl": 1800,
"content": "admin.example.com Phone: (800)-652-4786",
"state": false
}
"mailbox": "admin.example.com",
"id": 1852891,
"domain_id": 7341211,
"domain_name": "example.com",
"node_name": "rp",
"hostname": "rp.example.com",
"record_type": "RP",
"ttl": 1800,
"content": "admin.example.com Phone: (800)-652-4786",
"state": false
}
6
Delete a record
GET /v1/dns/record/delete/:id
Parameters
Name | Type | Description |
---|---|---|
:id | integer | The record id |
Delete the record with ID
1852895
which is an A record for subdomain name sub.example.com
:
curl -v https://api.dynu.com/v1/dns/record/delete/1852895 \
-H "Content-Type: application/json" \
-H "Authorization: Bearer <token>"
Response-H "Content-Type: application/json" \
-H "Authorization: Bearer <token>"
Responds with HTTP/1.1 200 OK on success. Returns true.
Email Service
Domains
1
List a list of domains
GET /v1/email/domains
Example
curl -v https://api.dynu.com/v1/email/domains \
-H "Content-Type: application/json" \
-H "Authorization: Bearer <token>"
Response-H "Content-Type: application/json" \
-H "Authorization: Bearer <token>"
Responds with HTTP/1.1 200 OK if successful, returns a list of domain names with email service properties.
[
{
"id": 1,
"user_id": 11,
"name": "example.com",
"token": "domain-token",
"state": "Complete",
"type": "FullServiceEmail",
"auto_renewal": true,
"anti_spam": false,
"etrn_host": null,
"etrn_port": 0,
"etrn_connection_security": null,
"etrn_retry_in terval": 0,
"catch_all_address":"someaddress@gmail.com",
"plus_addressing": false,
"plus_addressing_character": "+",
"expires_on": "2016-07-28T14:57:08",
"created_at": "2015-07-28T15:01:13",
"updated_at": "2015-09-10T13:03:15"
},
{
"id": 2,
"user_id": 11,
"name": "example1.com",
"token": "domain-token",
"state": "Complete",
"type": "SMTPOutboundRelay",
"auto_renewal": true,
"anti_spam": false,
"etrn_host": null,
"etrn_port": 0,
"etrn_connection_security": null,
"etrn_retry_in terval": 0,
"plus_addressing": false,
"plus_addressing_character": null,
"expires_on": "2017-01-23T18:24:41",
"created_at": "2016-01-23T18:01:27",
"updated_at": "2016-01-2 3T18:01:27"
},
{
"id": 3,
"user_id": 11,
"name": "example2.com",
"token": "domain-token",
"state": "Complete",
"type": "EmailStoreForward",
"auto_renewal": true,
"anti_spam": false,
"etrn_host": "mail.example2.com",
"etrn_port": 26,
"etrn_connection_security": "SSLTLS",
"etrn_retry_in terval": 10,
"plus_addressing": false,
"plus_addressing_character": null,
"expires_on": "2017-11-29T13:24:35",
"created_at": "2015-11-29T13:19:52",
"updated_at": "2016-01-09T0 3:49:20"
},
{
"id": 4,
"user_id": 11,
"name": "example3.com",
"token": "domain-token",
"state": "Complete",
"type": "EmailBackup",
"auto_renewal": true,
"anti_spam": false,
"etrn_host": "mail.example3.com",
"etrn_port": 2525,
"etrn_connection_security": "None",
"etrn_retry_in terval": 20,
"plus_addressing": false,
"plus_addressing_character": null,
"expires_on": "2017-11-29T13:21:44",
"created_at": "2015-11-29T13:21:35",
"updated_at": "2015-12-23T13:47:27"
},
{
"id": 5,
"user_id": 11,
"name": "example4.com",
"token": "domain-token",
"state": "Complete",
"type": "EmailForward",
"auto_renewal": true,
"anti_spam": true,
"etrn_host": null,
"etrn_port": 0,
"etrn_connection_security": null,
"etrn_retry_in terval": 0,
"plus_addressing": false,
"plus_addressing_character": null,
"expires_on": "2016-07-28T14:57:36",
"created_at": "2015-11-29T14:57:36",
"updated_at": "2015-12-23T14:26:39"
},
]
{
"id": 1,
"user_id": 11,
"name": "example.com",
"token": "domain-token",
"state": "Complete",
"type": "FullServiceEmail",
"auto_renewal": true,
"anti_spam": false,
"etrn_host": null,
"etrn_port": 0,
"etrn_connection_security": null,
"etrn_retry_in terval": 0,
"catch_all_address":"someaddress@gmail.com",
"plus_addressing": false,
"plus_addressing_character": "+",
"expires_on": "2016-07-28T14:57:08",
"created_at": "2015-07-28T15:01:13",
"updated_at": "2015-09-10T13:03:15"
},
{
"id": 2,
"user_id": 11,
"name": "example1.com",
"token": "domain-token",
"state": "Complete",
"type": "SMTPOutboundRelay",
"auto_renewal": true,
"anti_spam": false,
"etrn_host": null,
"etrn_port": 0,
"etrn_connection_security": null,
"etrn_retry_in terval": 0,
"plus_addressing": false,
"plus_addressing_character": null,
"expires_on": "2017-01-23T18:24:41",
"created_at": "2016-01-23T18:01:27",
"updated_at": "2016-01-2 3T18:01:27"
},
{
"id": 3,
"user_id": 11,
"name": "example2.com",
"token": "domain-token",
"state": "Complete",
"type": "EmailStoreForward",
"auto_renewal": true,
"anti_spam": false,
"etrn_host": "mail.example2.com",
"etrn_port": 26,
"etrn_connection_security": "SSLTLS",
"etrn_retry_in terval": 10,
"plus_addressing": false,
"plus_addressing_character": null,
"expires_on": "2017-11-29T13:24:35",
"created_at": "2015-11-29T13:19:52",
"updated_at": "2016-01-09T0 3:49:20"
},
{
"id": 4,
"user_id": 11,
"name": "example3.com",
"token": "domain-token",
"state": "Complete",
"type": "EmailBackup",
"auto_renewal": true,
"anti_spam": false,
"etrn_host": "mail.example3.com",
"etrn_port": 2525,
"etrn_connection_security": "None",
"etrn_retry_in terval": 20,
"plus_addressing": false,
"plus_addressing_character": null,
"expires_on": "2017-11-29T13:21:44",
"created_at": "2015-11-29T13:21:35",
"updated_at": "2015-12-23T13:47:27"
},
{
"id": 5,
"user_id": 11,
"name": "example4.com",
"token": "domain-token",
"state": "Complete",
"type": "EmailForward",
"auto_renewal": true,
"anti_spam": true,
"etrn_host": null,
"etrn_port": 0,
"etrn_connection_security": null,
"etrn_retry_in terval": 0,
"plus_addressing": false,
"plus_addressing_character": null,
"expires_on": "2016-07-28T14:57:36",
"created_at": "2015-11-29T14:57:36",
"updated_at": "2015-12-23T14:26:39"
},
]
2
Get a domain
Get a domain name with email service properties
Get /v1/email/get/:domain
Parameters
Name | Type | Description |
---|---|---|
:domain | string, integer | The domain name |
Get the domain
example.com
.
curl -v https://api.dynu.com/v1/email/get/example.com \
-H "Content-Type: application/json" \
-H "Authorization: Bearer <token>"
Response-H "Content-Type: application/json" \
-H "Authorization: Bearer <token>"
Responds with HTTP/1.1 200 OK if successful.returns the domain name with email service properties.
{
"id": 1,
"user_id": 11,
"name": "example.com",
"token": "domain-token",
"state": "Complete",
"type": "FullServiceEmail",
"auto_renewal": true,
"anti_spam": false,
"etrn_host": null,
"etrn_port": 0,
"etrn_connection_security": null,
"etrn_retry_in terval": 0,
"catch_all_address":"yexiaos@hotmail.com",
"plus_addressing": false,
"plus_addressing_character": "+",
"expires_on": "2016-07-28T14:57:08",
"created_at": "2015-07-28T15:01:13",
"updated_at": "2015-09-10T13:03:15"
}
"id": 1,
"user_id": 11,
"name": "example.com",
"token": "domain-token",
"state": "Complete",
"type": "FullServiceEmail",
"auto_renewal": true,
"anti_spam": false,
"etrn_host": null,
"etrn_port": 0,
"etrn_connection_security": null,
"etrn_retry_in terval": 0,
"catch_all_address":"yexiaos@hotmail.com",
"plus_addressing": false,
"plus_addressing_character": "+",
"expires_on": "2016-07-28T14:57:08",
"created_at": "2015-07-28T15:01:13",
"updated_at": "2015-09-10T13:03:15"
}
Accounts
1
Add an email account
POST /v1/email/email_account_add/:domain/:account
Parameters
Name | Type | Description |
---|---|---|
:account | string, integer | The email address |
admin@example.com
.
curl -v -X POST https://api.dynu.com/v1/email/email_account_add/example.com \
-d "{\"email_address\": [\"admin@example.com\",\"password\": \"somepassword\",\"state\": \"active\",\"use_forwarding\": \"true\", \"keep_original_messsage\": \"true\",\"forward_address\": \"thomas@gmail.com\" ]}" \
-H "Content-Type: application/json" \
-H "Authorization: Bearer <token>"
Response-d "{\"email_address\": [\"admin@example.com\",\"password\": \"somepassword\",\"state\": \"active\",\"use_forwarding\": \"true\", \"keep_original_messsage\": \"true\",\"forward_address\": \"thomas@gmail.com\" ]}" \
-H "Content-Type: application/json" \
-H "Authorization: Bearer <token>"
Responds with HTTP/1.1 200 OK if successful. Return the email account.
{
"id": 34,
"domain_id": 277,
"email_address": "admin@example.com",
"password":null,
"state": "Active",
"use_forwarding":true,
"keep_original_messsage": true,
"forward_address": "thomas@gmail.com",
}
"id": 34,
"domain_id": 277,
"email_address": "admin@example.com",
"password":null,
"state": "Active",
"use_forwarding":true,
"keep_original_messsage": true,
"forward_address": "thomas@gmail.com",
}
2
Get an email account
GET /v1/email/get/:account
Parameters
Name | Type | Description |
---|---|---|
:account | string, integer | The email address |
admin@example.com
.
curl -v https://api.dynu.com/v1/email/get/admin@example.com \
-H "Content-Type: application/json" \
-H "Authorization: Bearer <token>"
Response-H "Content-Type: application/json" \
-H "Authorization: Bearer <token>"
Responds with HTTP/1.1 200 OK if successful. Returns the email account.
{
"id": 34,
"domain_id": 277,
"email_address": "admin@example.com",
"password":null,
"state": "Active",
"use_forwarding":true,
"keep_original_messsage": true,
"forward_address": "thomas@gmail.com",
}
"id": 34,
"domain_id": 277,
"email_address": "admin@example.com",
"password":null,
"state": "Active",
"use_forwarding":true,
"keep_original_messsage": true,
"forward_address": "thomas@gmail.com",
}
3
List email accounts
GET /v1/email/email_accounts/:domain
Example
curl -v https://api.dynu.com/v1/email/email_accounts/example.com \
-H "Content-Type: application/json" \
-H "Authorization: Bearer <token>"
Response-H "Content-Type: application/json" \
-H "Authorization: Bearer <token>"
Responds with HTTP/1.1 200 OK if successful, returns a list of email accounts.
[
{
"id": 34,
"domain_id": 277,
"email_address": "admin@example.com",
"password":null,
"state": "Active",
"use_forwarding":true,
"keep_original_messsage": true,
"forward_address": "thomas@gmail.com",
},
{
"id": 35,
"domain_id": 277,
"email_address": "tom@example.com",
"password":null,
"state": "Inactive",
"use_forwarding":true,
"keep_original_messsage": true,
"forward_address": "tom@yahoo.com",
},
{
"id": 36,
"domain_id": 277,
"email_address": "amanda@example.com",
"password":null,
"state": "Active",
"use_forwarding":false,
"keep_original_messsage": false,
"forward_address": null,
}
]
{
"id": 34,
"domain_id": 277,
"email_address": "admin@example.com",
"password":null,
"state": "Active",
"use_forwarding":true,
"keep_original_messsage": true,
"forward_address": "thomas@gmail.com",
},
{
"id": 35,
"domain_id": 277,
"email_address": "tom@example.com",
"password":null,
"state": "Inactive",
"use_forwarding":true,
"keep_original_messsage": true,
"forward_address": "tom@yahoo.com",
},
{
"id": 36,
"domain_id": 277,
"email_address": "amanda@example.com",
"password":null,
"state": "Active",
"use_forwarding":false,
"keep_original_messsage": false,
"forward_address": null,
}
]
4
Remove an email account
Get /v1/email/email_account_remove/:account
Parameters
Name | Type | Description |
---|---|---|
:account | string, integer | The email address |
Remove the email account
admin@example.com
.
curl -v https://api.dynu.com/v1/email/email_account_remove/admin@example.com \
-H "Content-Type: application/json" \
-H "Authorization: Bearer <token>"
Response-H "Content-Type: application/json" \
-H "Authorization: Bearer <token>"
Responds with HTTP/1.1 200 OK if successful. Returns the rest of email accounts for that domain name.
[
{
"id": 35,
"domain_id": 277,
"email_address": "tom@example.com",
"password":null,
"state": "Inactive",
"use_forwarding":true,
"keep_original_messsage": true,
"forward_address": "tom@yahoo.com",
} ,
{
"id": 36,
"domain_id": 277,
"email_address": "amanda@example.com",
"password":null,
"state": "Active",
"use_forwarding":false,
"keep_original_messsage": false,
"forward_address": null,
}
]
{
"id": 35,
"domain_id": 277,
"email_address": "tom@example.com",
"password":null,
"state": "Inactive",
"use_forwarding":true,
"keep_original_messsage": true,
"forward_address": "tom@yahoo.com",
} ,
{
"id": 36,
"domain_id": 277,
"email_address": "amanda@example.com",
"password":null,
"state": "Active",
"use_forwarding":false,
"keep_original_messsage": false,
"forward_address": null,
}
]
5
Enable an email account
GET /v1/email_account_enable/:account
ExampleEnable email account amanda@example.com
curl -v https://api.dynu.com/v1/email/email_account_enable/amanda@example.com \
-H "Content-Type: application/json" \
-H "Authorization: Bearer <token>"
Response-H "Content-Type: application/json" \
-H "Authorization: Bearer <token>"
Responds with HTTP/1.1 200 OK if successful, returns the email account.
{
"id": 36,
"domain_id": 277,
"email_address": "amanda@example.com",
"password":null,
"state": "Active",
"use_forwarding":false,
"keep_original_messsage": false,
"forward_address": null,
}
"id": 36,
"domain_id": 277,
"email_address": "amanda@example.com",
"password":null,
"state": "Active",
"use_forwarding":false,
"keep_original_messsage": false,
"forward_address": null,
}
6
Disable an email account
GET /v1/email_account_disable/:account
ExampleDisable email account amanda@example.com
curl -v https://api.dynu.com/v1/email/email_account_disable/amanda@example.com \
-H "Content-Type: application/json" \
-H "Authorization: Bearer <token>"
Response-H "Content-Type: application/json" \
-H "Authorization: Bearer <token>"
Responds with HTTP/1.1 200 OK if successful, returns the email account.
{
"id": 36,
"domain_id": 277,
"email_address": "amanda@example.com",
"password":null,
"state": "Inactive",
"use_forwarding":false,
"keep_original_messsage": false,
"forward_address": null,
}
"id": 36,
"domain_id": 277,
"email_address": "amanda@example.com",
"password":null,
"state": "Inactive",
"use_forwarding":false,
"keep_original_messsage": false,
"forward_address": null,
}